Thymeleaf 是⼀种类似于 JSP 的动态⽹⻚技术
1. Thymeleaf简介
JSP 必须依赖Tomcat运⾏,不能直接运⾏在浏览器中
HTML可以直接运⾏在浏览器中,但是不能接收控制器传递的数据
Thymeleaf是⼀种既保留了HTML的后缀能够直接在浏览器运⾏的能⼒、⼜实现了JSP显示动态数据的功能——静能查看⻚⾯效果、动则可以显示数据
2. Thymeleaf应用
2.1 添加thymelea的starter
在springboot不需要指定版本,内置有版本
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
2.2 创建thymelea模板
Thymeleaf 模板就是 HTML ⽂件
SpringBoot 应⽤中 resources\templates ⽬录就是⽤来存放⻚⾯模板的
2.2.1重要说明:
static ⽬录下的资源被定义静态资源, SpringBoot 应⽤默认放⾏;如果将 HTML ⻚⾯创建 static ⽬录是可以 直接访问的
templates ⽬录下的⽂件会被定义为动态⽹⻚模板, SpringBoot 应⽤会拦截 templates 中定义的资源;如果将 HTML⽂件定义在 templates ⽬录,则必须通过控制器跳转访问。
在 templates 创建 HTML ⻚⾯模板
创建 PageController ,⽤于转发允许 " 直接访问 " 的⻚⾯请求
2.2.2简单应用:
@Controller
@RequestMapping("/book")
public class BookController {
@RequestMapping("/query")
public String queryBook(int bookId, Model model){
Book book = new Book(bookId, "java", "亮哥");
model.addAttribute("book",book);
return "test";
}
}
test.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
test
<hr/>
<label th:text="${book.bookName}">图书名称</label>
</body>
</html>
在浏览器中输入:
http://localhost:8080/book/query?bookId=1
3 Thymeleaf基本语法
如果要在 thymeleaf 模板中获取从控制传递的数据,需要使⽤ th 标签
3.1在thymeleaf模板⻚⾯引⼊th标签的命名空间
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
test
<hr/>
<label th:text="${book.bookName}">图书名称</label>
</body>
</html>
3.2 th:text
在⼏乎所有的 HTML 双标签都可以使⽤ th:text 属性,将接收到的数据显示在标签的内容中
@Controller
@RequestMapping("/book")
public class BookController {
@RequestMapping("/query")
public String queryBook(int bookId, Model model){
Book book = new Book(bookId, "java", "亮哥");
List<Book> books = new ArrayList<>();
books.add(new Book(1,"java","亮哥1"));
books.add(new Book(2,"html","亮哥2"));
books.add(new Book(3,"c++","亮哥3"));
books.add(new Book(4,"python","亮哥4"));
//简单类型
model.addAttribute("price",3.22);
//字符串
model.addAttribute("str","Long Long ago...");
//对象
model.addAttribute("book",book);
//集合
model.addAttribute("books",books);
return "test";
}
}
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org" xmlns="http://www.w3.org/1999/html">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
价格:<label th:text="${price}"></label> </br>
字符串:<div th:text="${str}"></div>
<p th:text="${book.bookName}">图书名称:</p>
</body>
</html>
3.3 th:inline内联
3.3.1 HTML 内联
<p th:inline="text">图书名称:[[${book.bookName}]]</p>
3.3.2 CSS内联
model.addAttribute("color","orange");
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org" xmlns="http://www.w3.org/1999/html">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style type="text/css" th:inline="css">
.style1{
color: [[${color}]];
}
</style>
</head>
<body>
价格:<label th:text="${price}"></label> </br>
字符串:<div th:text="${str}"></div>
<p th:inline="text" class="style1">图书名称:[[${book.bookName}]]</p>
</body>
</html>
重启刷新:变色成功
3.3.3 javaAcript内联
和css内联差不多
<script type="css/javascript" th:inline="javascript">
</script>
3.3.4 th:object和*
外层有th:object 内层就可以用*代表上层对象
<div th:object="${book}">
<p th:text="*{bookId}"></p>
<p th:text="*{bookName}"></p>
<p th:text="*{bookAuthor}"></p>
</div>
4. 流程控制
4.1 th:each 循环
<table style="width: 600px" border="1" cellspacing="0">
<caption>图书信息列表</caption>
<thead>
<tr>
<th>图书ID</th>
<th>图书名称</th>
<th>图书作者</th>
</tr>
</thead>
<tbody>
<tr th:each="b:${books}">
<td th:text="${b.bookId}"></td>
<td th:text="${b.bookName}"></td>
<td th:text="${b.bookAuthor}"></td>
</tr>
</tbody>
</table>
4.2 分支
4.2.1 th:if 如果条件不成立,则不显示此标签
<td th:if="${b.bookPrice}>40" style="color:red">太贵!!!</td>
<td th:if="${b.bookPrice}<=40" style="color:green">推荐购买</td>
4.2.2 th:switch和th:case
<td th:switch="${b.bookPrice}/10">
<label th:case="3">建议购买</label>
<label th:case="4">价格合理</label>
<label th:case="*">价格不合理</label>
</td>
<td th:switch="${user.gender}">
<label th:case="M">男</label>
<label th:case="F">⼥</label>
<label th:case="*">性别不详</label>
</td>
5. 碎片使用
5.1 碎片的概念
碎⽚,就是 HTML ⽚段,我们可以将多个⻚⾯中使⽤的相同的 HTML 标签部分单独定义,然后通过 th:include 可以在HTML ⽹⻚中引⼊定义的碎⽚
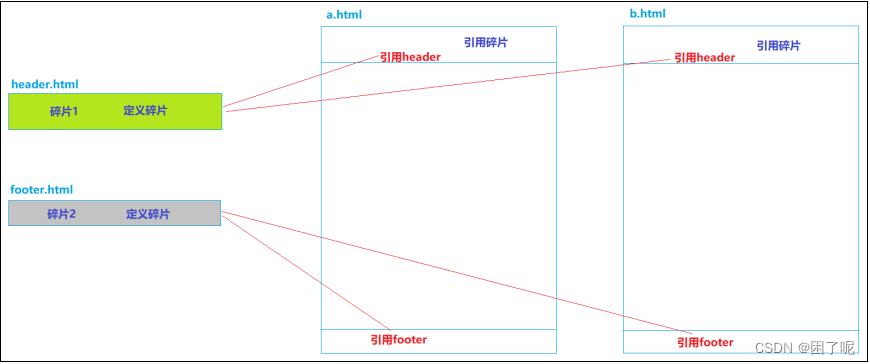
5.2 碎片使用案例
5.2.1 定义碎片 th:fragment
header.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div th:fragment="fragment1" style="width: 100%; height: 80px;background: deepskyblue; color:white; font-size: 25px; font-family:⽂鼎霹雳体">
头部测试,(~ ̄▽ ̄)~666!!
</div>
</body>
</html>
footer.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div th:fragment="fragment2" style="width: 100%; height: 30px;background: lightgray; color:white; font-size: 16px;">
尾部测试,(~ ̄▽ ̄)~666!!
</div>
</body>
</html>
5.2.2 引用碎片 th:include和th:replace
a.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div th:include="header::fragment1"></div>
<div style="width: 100%;height: 500px">
定义内容
</div>
<div th:include="footer::fragment2"></div>
</body>
</html>
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<!-- <div th:include="header::fragment1"></div>-->
<div th:replace="header::fragment1"></div>
<div style="width: 100%;height: 500px">
定义内容
</div>
<div th:include="footer::fragment2"></div>
<div th:replace="footer::fragment2"></div>
</body>
</html>
版权声明:本文为l472620667原创文章,遵循CC 4.0 BY-SA版权协议,转载请附上原文出处链接和本声明。