sql中的while循环
The SQL While loop is used to repeatedly execute a certain piece of SQL script.
SQL While循环用于重复执行某段SQL脚本。
This article covers some of the basic functionalities of the SQL While loop in Microsoft SQL Server, with the help of examples.
本文通过示例介绍了Microsoft SQL Server中SQL While循环的一些基本功能。
SQL While循环语法 (SQL While loop syntax)
The syntax of the SQL While loop is as follows:
SQL While循环的语法如下:
WHILE condition
BEGIN
//SQL Statements
END;
The while loop in SQL begins with the WHILE keyword followed by the condition which returns a Boolean value i.e. True or False.
SQL中的while循环以WHILE关键字开头,后跟返回布尔值(即True或False)的条件。
The body of the while loop keeps executing unless the condition returns false. The body of a while loop in SQL starts with a BEGIN block and ends with an END block.
除非条件返回false,否则while循环的主体将继续执行。 SQL中while循环的主体以BEGIN块开始,以END块结束。
一个简单的示例:使用SQL While循环打印数字 (A simple example: Printing numbers with SQL While loop)
Let’s start with a very simple example where we use a SQL While loop to print the first five positive integer values:
让我们从一个非常简单的示例开始,在该示例中,我们使用SQL While循环打印前五个正整数值:
DECLARE @count INT;
SET @count = 1;
WHILE @count<= 5
BEGIN
PRINT @count
SET @count = @count + 1;
END;
In the script above, we first declare an integer type variable @count and set its value to 5.
在上面的脚本中,我们首先声明一个整数类型变量@count并将其值设置为5。
Next, we execute a While loop which checks if the value of the @count variable is less than or equals to 5. If the @count variable has a value less than or equals to 5, the body of the loop executes, and the current value of the @count variable is printed on the console.
接下来,我们执行一个While循环,该循环检查@count变量的值是否小于或等于5。如果@count变量的值小于或等于5,则执行循环主体,并执行当前操作。 @count变量的值将打印在控制台上。
In the next line, the value of the @count variable is incremented by 1. The While loop keeps executing until the value of the @count variable becomes greater than 5. Here is the output:
在下一行,@count变量的值增加1。While循环一直执行,直到@count变量的值大于5。这是输出:
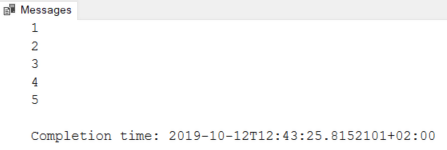
使用SQL While循环插入记录 (Inserting records with SQL While loop)
Let’s now see how the SQL While loop is used to insert dummy records in a database table.
现在,让我们看看如何使用SQL While循环在数据库表中插入伪记录。
For this we need a simple database “CarShop”:
为此,我们需要一个简单的数据库“ CarShop”:
CREATE DATABASE CarShop
We will create one table i.e. Cars within the CarShop database. The Cars table will have three columns Id, Name and Price. Execute the following script:
我们将在CarShop数据库中创建一个表,即Cars。 汽车表将具有三列ID,名称和价格。 执行以下脚本:
USE CarShop
CREATE TABLE Cars
(
Id INT PRIMARY KEY IDENTITY(1,1),
Name VARCHAR (50) NOT NULL,
Price INT
)
Let’s now use the While loop in SQL to insert 10 records in the Cars table. Execute the following script:
现在,让我们使用SQL中的While循环在Cars表中插入10条记录。 执行以下脚本:
DECLARE @count INT;
SET @count = 1;
WHILE @count<= 10
BEGIN
INSERT INTO Cars VALUES('Car-'+CAST(@count as varchar), @count*100)
SET @count = @count + 1;
END;
In the script above, we again declare a variable @count and initialize it with 1. Next, a while loop is executed until the value of the @count variable becomes greater than 10, which means that the while loop executes 10 times.
在上面的脚本中,我们再次声明变量@count并用1对其进行初始化。接下来,执行while循环,直到@count变量的值大于10,这意味着while循环执行10次。
In the body of the while loop, the INSERT query is being used to insert one record into the Cars table. For the Name column, the value of the @count variable is appended with the string Car-
. For the Price column of the Cars table, the value of the @count variable is multiplied by 100.
在while循环的主体中,使用INSERT查询将一条记录插入Cars表中。 对于“名称”列,@ count变量的值后面附加字符串Car-
。 对于Cars表的Price列,@ count变量的值乘以100。
Now if you select all the records from the Cars table with the “SELECT * FROM Cars” query, you should see the following output:
现在,如果使用“ SELECT * FROM Cars”查询从Cars表中选择所有记录,则应该看到以下输出:
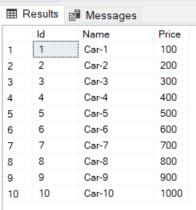
使用SQL While循环实现分页 (Implementing paging with SQL While loop)
The while loop can also be used to implement paging. Paging refers to displaying a subset of records from a data table at any particular time.
while循环还可用于实现分页。 分页是指在任何特定时间显示数据表中记录的子集。
In the following script, the while loop will be used to select two records from the Cars table at a time. The selected records are then displayed in the console output:
在以下脚本中,while循环将用于一次从Cars表中选择两个记录。 然后,所选记录将显示在控制台输出中:
DECLARE @count INT
DECLARE @limit INT;
SET @count = 0
SET @limit = 2;
WHILE @count< 10
BEGIN
SELECT * FROM Cars
ORDER BY Id
OFFSET @count ROWS
FETCH NEXT @limit ROWS ONLY
SET @count = @count + 2;
END;
In the script above, we initialize two variables i.e. @count and @limit. The initial values for the @count and @limit variables are 0 and 2, respectively. The while loop executes while the value of the @count variable remains less than 10.
在上面的脚本中,我们初始化了两个变量,即@count和@limit。 @count和@limit变量的初始值分别为0和2。 当@count变量的值保持小于10时,将执行while循环。
Inside the while loop, the OFFSET clause is used to skip the first N rows of the Cars table. The FETCH NEXT clause fetches the next N records.
在while循环内,OFFSET子句用于跳过Cars表的前N行。 FETCH NEXT子句获取接下来的N条记录。
In the first iteration, the value of OFFSET will be 0 since @count is 0, the first two records will be displayed. In the second iteration, since the @count variable will have the value 2, the first two records will be skipped and the records 3 and 4 will be retrieved.
在第一次迭代中,由于@count为0,因此OFFSET的值为0,将显示前两个记录。 在第二次迭代中,由于@count变量的值为2,因此将跳过前两个记录,并检索记录3和4。
In this way, all the records from the Cars table will be retrieved in sets of two. The output is as follows:
这样,Cars表中的所有记录将以两个为一组进行检索。 输出如下:
In the output, you can see all the records from the Cars table, printed in sets of two on the console.
在输出中,您可以查看Cars表中的所有记录,这些记录以两个一组的形式打印在控制台上。
CONTINUE和BREAK语句 (The CONTINUE and BREAK statements)
The CONTINUE statement is used to shift the control back to the start of a while loop in SQL. The BREAK statement is used to terminate the loop.
CONTINUE语句用于将控件移回SQL中的while循环的开始。 BREAK语句用于终止循环。
The following script shows how to use the CONTINUE statement inside a while loop to print the first five positive even integers:
以下脚本显示了如何在while循环中使用CONTINUE语句打印前五个正偶数整数:
DECLARE @count INT;
DECLARE @mod INT;
SET @count = 1;
WHILE @count<= 10
BEGIN
set @mod = @count % 2
IF @mod = 1
BEGIN
SET @count = @count + 1;
CONTINUE
END
PRINT @count
SET @count = @count + 1;
END;
In the script above, the while loop executes until the value of the @count variable remains less than or equal to 10. The initial value of the @count variable is 1.
在上面的脚本中,执行while循环,直到@count变量的值保持小于或等于10。@count变量的初始值为1。
In the body of the loop, the value of the remainder of the @count divided by 2 is stored in the @mod variable. If the value of the @count variable is odd, the remainder will be 1, and if the remainder is 0, the CONTINUE statement is used to shift the control back to the start of the while loop and the value of the @count variable is not printed.
在循环的主体中,@ count的余数除以2将存储在@mod变量中。 如果@count变量的值为奇数,则余数为1,如果余数为0,则使用CONTINUE语句将控件移回到while循环的开始,并且@count变量的值为不打印。
Otherwise, the value of the @count variable is printed on the console. Here is the output of the above script:
否则,@ count变量的值将打印在控制台上。 这是上面脚本的输出:
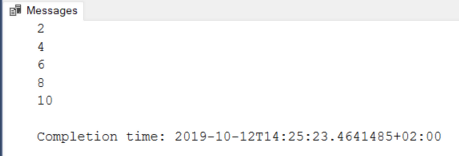
The following example demonstrates the use of a BREAK statement. The while loop in the following script will terminate after printing the first five integers:
下面的示例演示BREAK语句的用法。 在打印前五个整数后,以下脚本中的while循环将终止:
DECLARE @count INT;
SET @count = 1;
WHILE @count<= 10
BEGIN
IF @count > 5
BEGIN
BREAK
END
PRINT @count
SET @count = @count + 1;
END;
结论 (Conclusion)
If you want to repeatedly execute a particular SQL script, the SQL While loop is the way to go. The article explains how to use the SQL While loop in Microsoft SQL Server to perform a variety of tasks ranging from record insertion to pagination.
如果要重复执行特定SQL脚本,则可以使用SQL While循环。 本文介绍了如何在Microsoft SQL Server中使用SQL While循环执行从记录插入到分页的各种任务。
翻译自: https://www.sqlshack.com/sql-while-loop-understanding-while-loops-in-sql-server/
sql中的while循环